"The value of a man should be seen in what he gives and not in what he is able to receive"
― Albert Einstein ―
What is Common Language Runtime (CLR)?
It’s a virtual machine component of .NET framework that manages your compiled code. When you write a C#, VB, or any other .net language in Visual Studio and build your project, the compiler takes that code and converts the code into a compiled code. The complied code is also known as assembly code. This complied code is managed by CLR. It’s a responsibility of CLR to make sure that code can run on any windows platform regardless of hardware or operating system. The CLR easily manage the code for you by making sure that it runs without any issues and objects that are beings used in the program are removed from the memory when they are no longer needed. The CLR also allows different programs to share the common classes that were written in different .Net languages.
Json formatted content using List Object, Class [ string and string[] accessors]
In this article I will show you how to format the following Json data using list<object>, Class that includes String properties and string[] array accessor methods.
The output result must be in the following format.
[{"Name":"Asad","Description":"Student of Islam","Keywords":["value","value1","value3"]}]
The below diagram shows how List<object>, String Properties and sting[] in a class will produce the above required format.
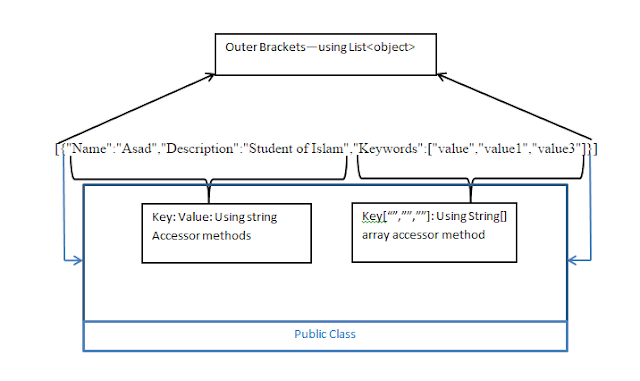
public class jsonFormatting
{
public string GetData()
{
Person p = new Person
{
Keywords = new[] { "value","value1","value3" },
};
p.Name = "Asad";
p.Description = "Student of Islam";
List<object> people = new List<object>();
people.Add(p);
return Newtonsoft.Json.JsonConvert.SerializeObject(people);
}
}
public class Person
{
public string Name { get; set; }
public string Description { get; set; }
public string[] Keywords { get; set; }
}
The output result must be in the following format.
[{"Name":"Asad","Description":"Student of Islam","Keywords":["value","value1","value3"]}]
The below diagram shows how List<object>, String Properties and sting[] in a class will produce the above required format.
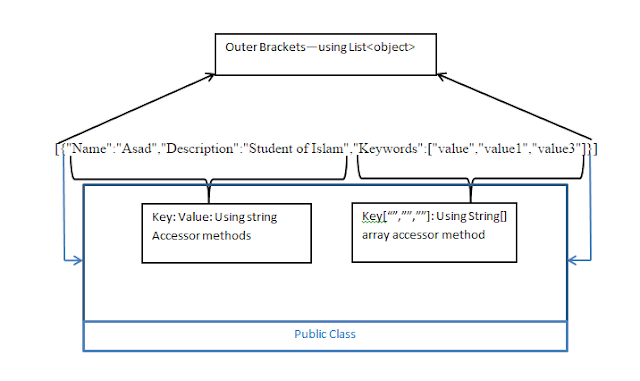
public class jsonFormatting
{
public string GetData()
{
Person p = new Person
{
Keywords = new[] { "value","value1","value3" },
};
p.Name = "Asad";
p.Description = "Student of Islam";
List<object> people = new List<object>();
people.Add(p);
return Newtonsoft.Json.JsonConvert.SerializeObject(people);
}
}
public class Person
{
public string Name { get; set; }
public string Description { get; set; }
public string[] Keywords { get; set; }
}
Subscribe to:
Posts (Atom)