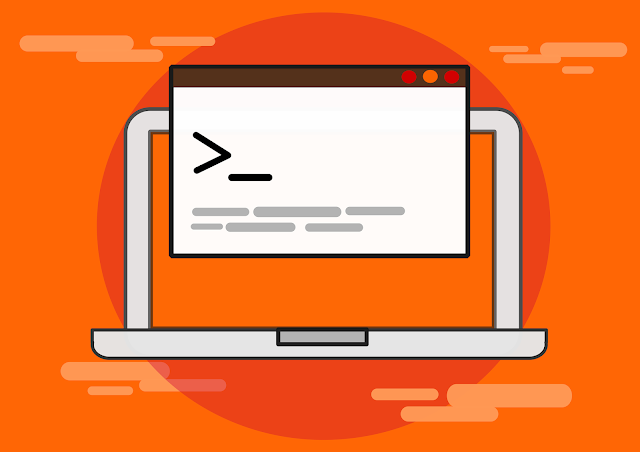 |
Installing MySQL on Ubuntu 18.04 |
Installing MySQL on Ubuntu 18.04
To install MySQL on your Ubuntu server follow the steps below:
Update the apt package index by typing:
sudo apt update
Install MySQL
- sudo apt install mysql-server
- Once the installation is completed, the MySQL service will start automatically. To check If MySQL server is running, type: sudo systemctl status mysql
mysql.service - MySQL Community Server
Loaded: loaded (/lib/systemd/system/mysql.service; enabled; vendor preset: en
Active: active (running) since Sun 2020-05-03 15:18:28 EDT; 1min 26s ago
Main PID: 4113 (mysqld)
Tasks: 27 (limit: 4588)
CGroup: /system.slice/mysql.service
└─4113 /usr/sbin/mysqld --daemonize --pid-file=/run/mysqld/mysqld.pid
Secure your MySQL
MySQL server package already has the command that can help us to secure our MSSQL.
Run the script by typing:
sudo mysql_secure_installation
VALIDATE PASSWORD PLUGIN can be used to test passwords
and improve security. It checks the strength of password
and allows the users to set only those passwords which are
secure enough. Would you like to setup VALIDATE PASSWORD plugin?
Enter YES
There are three levels of password validation policy:
LOW Length >= 8
MEDIUM Length >= 8, numeric, mixed case, and special characters
STRONG Length >= 8, numeric, mixed case, special characters and dictionary file
Please enter 0 = LOW,
1 = MEDIUM and 2 = STRONG:
1
Please set the password for root here.
New password:
Re-enter new password:
Estimated strength of the password: 100
Do you wish to continue with the password provided?(Press y|Y for Yes, any other key for No) :
Y
Remove anonymous users? (Press y|Y for Yes, any other key for No) :Y
Disallow root login remotely? (Press y|Y for Yes, any other key for No)
N
Remove test database and access to it? (Press y|Y for Yes, any other key for No) N
Reload privilege tables now? (Press y|Y for Yes, any other key for No)
Y
... skipping.
Reloading the privilege tables will ensure that all changes
made so far will take effect immediately.
Reload privilege tables now? (Press y|Y for Yes, any other key for No) :
Y
Success.
All done!
Login to SQL
sudo mysql
If you want to configure phpMyAdmin then change the authentication method from auth_socket to mysql_native_password.
ALTER USER 'root'@'localhost' IDENTIFIED WITH mysql_native_password BY 'Your password';
GRANT ALL PRIVILEGES ON *.* TO 'youruser'@'localhost' IDENTIFIED BY 'yourpassword';
OK let's run the following command: FLUSH PRIVILEGES This will tell the server to reload the grant tables and load your new changes.
SELECT user,authentication_string,plugin,host FROM mysql.user;
You will notice that it has your root user and a new user with "mysql_native_pa " not "auth_socket "
you can login with the following command if you already enabled password authentication.
you can login with the following command if you already enabled password authentication.
mysql -u root -p
OK you can type Quit so we can logout from SQL and install phpMyAdmin
It's about time to install phpMyAdmin to start managing your mysql instance on your Ubunut machine.
Type the following command: sudo apt install phpmyadmin php-mbstring php-gettext
 |
Enter on this screen. |
 |
Select Yes and Press Enter |
 |
Enter PHP Admin Password |
http://SERVER_IP/phpmyadmin (where SERVER_IP is the IP address of your hosting server) and log in.
My phpMyAdmin page is not displaying
 |
My phpMyAdmin page is not displaying |
If you are unable to bring up the phpmyadmin website then use the below instructions.
Open your apache2.conf in your editor.
sudo nano /etc/apache2/apache2.conf
Add the following line to the end of the file.
Include /etc/phpmyadmin/apache.conf
Then restart apache
sudo nano /etc/apache2/apache2.conf